How do I save an image from DALL-E?
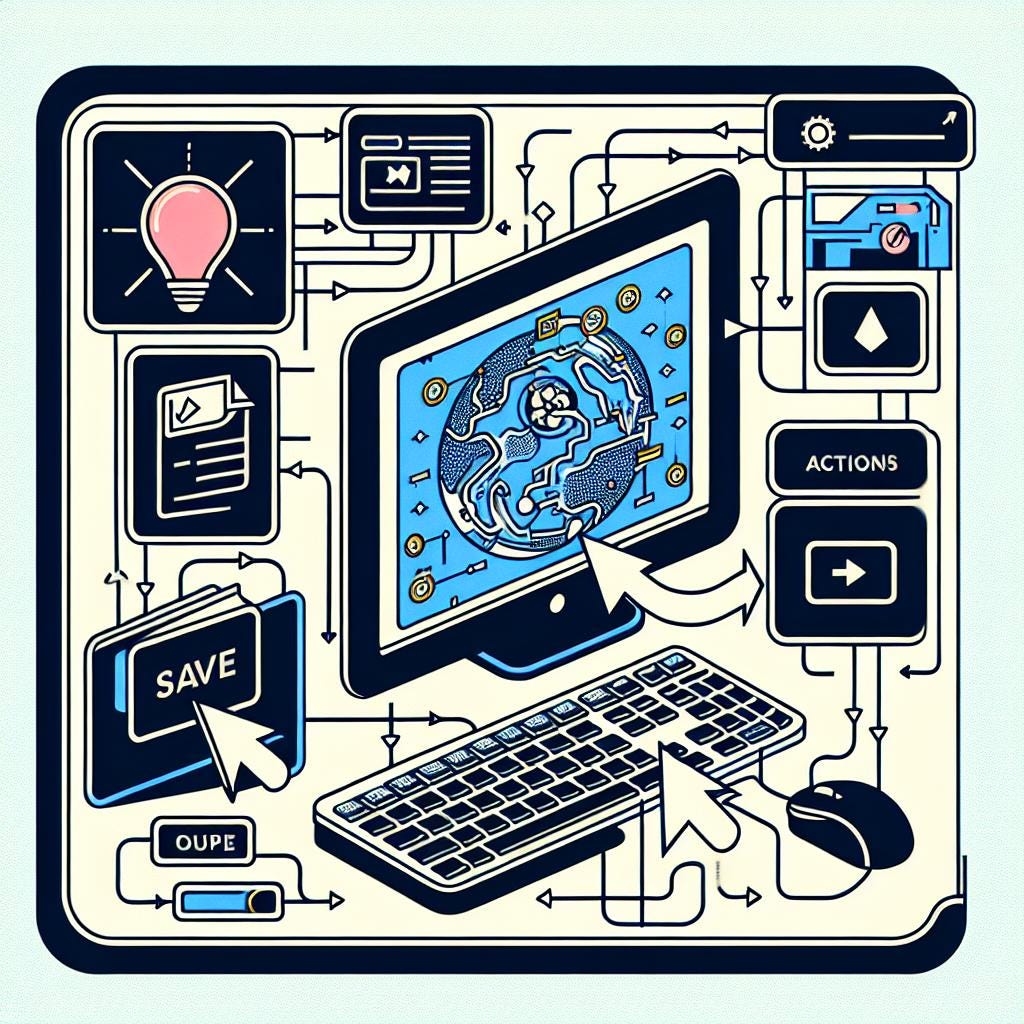
In recent years, DALL-E, an artificial intelligence model developed by OpenAI, has captivated the tech community with its ability to generate images from textual descriptions. This ground-breaking technology has opened new avenues for creativity, but it also presents intriguing challenges in terms of efficiently handling and storing the generated images. This article delves into a practical solution for saving images generated by DALL-E, particularly focusing on a Python-based approach encompassing image processing and storage optimization techniques.
Preparing the Environment
Before diving into the code, ensure that your environment is ready. You will need Python installed on your system along with the following libraries: PIL (Pillow), requests, and base64. These are essential for image processing and web requests handling. If you’re working within a custom application context (as the sample code suggests), you’ll also need access to your application modules for storage and configurations.
Fetching and Processing the Image
To start, let’s look at the process of fetching an image generated by DALL-E and converting it into a more storage-friendly format. The function process_image_to_base64 accomplishes this by performing the following steps:
1. Downloading the Image: Using the `requests` library, the image is fetched from a provided URL.
2. Converting to JPEG: To conserve space, the image is converted to a JPEG format using the `PIL` library, which can significantly reduce the file size with minimal loss of quality.
3. Encoding to Base64: The JPEG image is then encoded to a Base64 string, making it convenient to store or transmit as text.
import base64
import io
import requests
from PIL import Image
def process_image_to_base64(url):
response = requests.get(url)
image_bytes = io.BytesIO(response.content)
img = Image.open(image_bytes)
jpeg_image = io.BytesIO()
img.save(jpeg_image, format='JPEG')
jpeg_image.seek(0)
base64_string = base64.b64encode(jpeg_image.read()).decode('utf-8')
return "data:image/jpeg;base64," + base64_string
Generating and Storing the Image
With the process_image_to_base64 function ready, the next step is to generate an image from DALL-E and store it efficiently. This process is encapsulated in the createDalleImage function, which performs the following operations:
1. Making the Request to DALL-E: This involves sending a properly formatted JSON request to the DALL-E API. The request includes the user’s prompt, desired image size, quality, and style.
2. Handling the Response: Upon receiving the generated image’s URL, it’s converted to a Base64 string via `process_image_to_base64`.
4. Storing the Image: Finally, the Base64-encoded image is stored using the application’s storage system.
def createDalleImage(prompt, size="1024x1024", quality='standard', style='vivid'):
headers = {
'Authorization': f'Bearer {OPENAI_API_KEY}',
'Content-Type': 'application/json'
}
body = {
"model": "dall-e-3",
"prompt": prompt,
'n': 1,
"size": size,
"response_format": 'url',
"quality": quality,
"style": style
}
res = requests.post('https://api.openai.com/v1/images/generations', headers=headers, json=body)
image = res.json().get('data', [{}])[0]
image['url'] = process_image_to_base64(image['url'])
saveImage(image)
Key Considerations
The methodology presented here addresses several critical aspects of handling AI-generated images:
Efficiency: Converting images to JPEG and encoding them in Base64 can significantly reduce storage and bandwidth requirements.
Scalability: By encapsulating functionality into reusable functions, this approach can easily scale to accommodate higher volumes of image generation and processing.
Conclusion
Saving images from DALL-E efficiently requires careful consideration of format, size, and storage methods. The Python-based approach detailed in this article offers a structured process for downloading, processing, and storing images that balances quality with efficiency. As AI-generated content continues to reshape the digital landscape, mastering these techniques will be invaluable for developers and companies looking to leverage this technology to its fullest potential.